How to Simulate GraphQL APIs (And How We Do It)
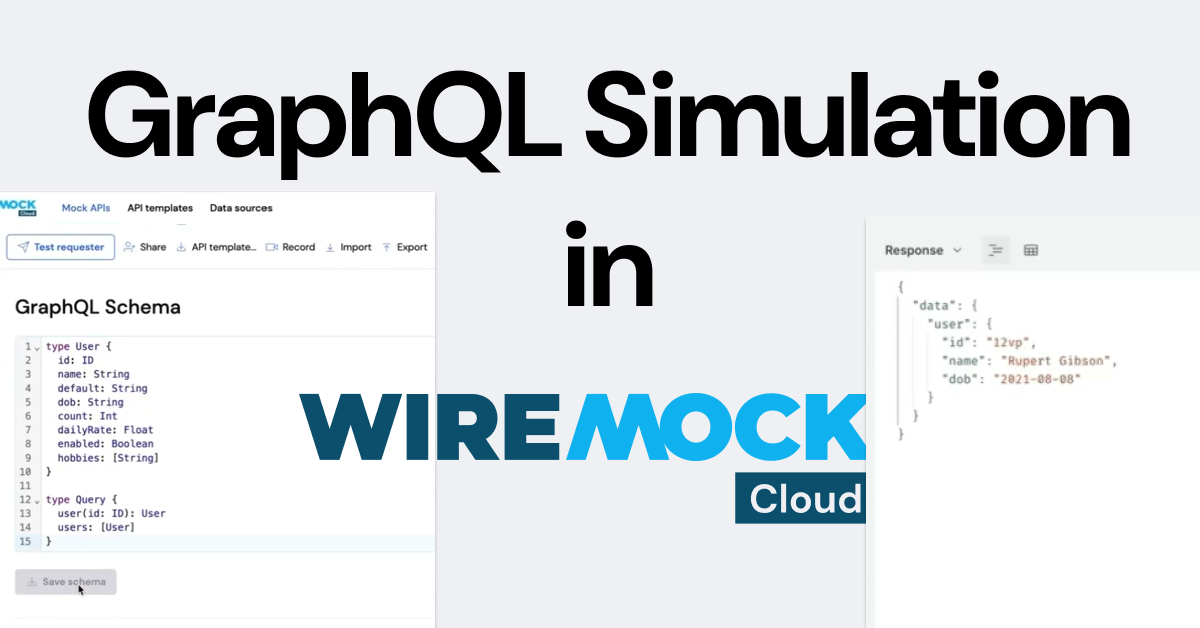
GraphQL is great if you’re looking for a flexible backend to support multiple front ends - but that same flexibility makes it a bit of a PITA when it comes to mocking. When your queries can be structured in countless ways and your schema keeps changing, traditional mocking approaches can quickly become frustrating time sinks.
In this article, we'll walk through what makes GraphQL APIs different from a simulation perspective, and show you how we designed our solution in a way that’s meant to be straightforward enough to start using immediately, while also enabling you to layer more advanced capabilities such as statefulness as you need them.
When Would You Simulate GraphQL APIs?
GraphQL offers a flexible query approach that solves many common challenges with REST APIs. Unlike REST, it lets clients request exactly what they need in a single query. This works well for teams with multiple frontends (web, mobile, internal tools) sharing a backend: each client gets everything it needs in one go instead of making multiple round-trips to different endpoints. This cuts down latency and makes for a better user experience, especially on mobile networks where connection overhead matters, and can also be simpler for developers to work with.
GraphQL federation takes these benefits further by combining multiple GraphQL services (subgraphs) into one cohesive API (supergraph). Teams can own their services independently while clients query across boundaries as if using a single API. This allows you to scale development across teams while maintaining a consistent API surface.
An Example
Here’s a (slightly modified) example we saw with one of our enterprise clients. Let’s say you’re building a travel app displaying booking information. One team is building a "manage booking" page that needs to show flight details, hotel information, car rentals, and activity reservations. With REST, you'd need several API calls, e.g.:
Each call adds latency, creates another potential failure point, and complicates error handling. You would also need to pass an identifier between queries, which may or may not work with all the different endpoints you’re using. GraphQL lets you request everything in one query:
But what happens when your frontend team wants to, e.g., add hotel room images to the booking page, and that service isn't ready yet? This is where you’ll need some kind of mock (assuming you don’t want developers twiddling their thumbs while waiting for that API to be ready). If you can simulate the GraphQL service or the entire federated graph, frontend developers building the booking management page can keep working without waiting for the backend. And if built correctly, this simulation should provide consistent responses matching the expected schema, allowing teams to build and test UI components independently.
This is far from a theoretical scenario, as in larger organizations you will often find different teams, each with their own roadmap and priorities, owning different services. The team building the booking page may control some data but the rest will depend on microservices that may or may not have test-ready APIs.
Why Mocking GraphQL is Harder Than Mocking REST
There are several reasons that mocking GraphQL is a distinct - and frustrating problem:
Less predictable responses make mocking more complicated. Mocking REST APIs is not exactly a solved problem, but it’s in a far more mature state than GraphQL. With REST, you have fixed endpoints with predictable payloads, which you can record at clear points in your tests, and you're off to the races. The limited request patterns make simulation relatively straightforward, at least for simpler use cases.
This is not the case with GraphQL. Instead of multiple endpoints with fixed shapes, you have a single endpoint where clients dictate exactly what data they want and how they want it structured. This is a headache when you’re trying to predict responses.
A client might request user { id name } while another needs user { id email orders { items { product price } } }. The variety creates an explosion of possibilities. In federated setups, this complexity multiplies as queries span multiple subgraphs with their own evolving schemas.
Current tools are often too involved to be useful. Most existing GraphQL mocking tools leave you with poor options. Some generate random data based solely on schema types, which are technically "valid" but disconnected from realistic business logic or edge cases. Others require excessive setup work - manually defined resolvers, code for each query pattern, and an ever-expanding catalog of special cases. Tools like Microcks involve workflows so complex they undermine the very point of mocking: rapid, flexible development.
Less mature ecosystem. For REST APIs, developers have a wide range of options from simple libraries to sophisticated API simulation platforms (ahem). Meanwhile, GraphQL teams are left cobbling together custom solutions or battling with tools where GraphQL support feels like an afterthought. Try simulating an error condition or a response that varies based on query variables, and these tools often fall short.
You're stuck choosing between sinking hours into custom mock code (defeating the purpose of using a tool) or settling for oversimplified mocks that leave gaps in your testing. Neither option is ideal for obvious reasons...
Simulating GraphQL in WireMock
WireMock makes GraphQL mocking simple: start with a schema, get a working mock immediately, and build more advanced capabilities as you need them. You can create your first functional mock in minutes without writing any resolvers upfront. WireMock also supports federation, handling both individual subgraphs and entire federated supergraphs.
Instantly launch your mock from a GraphQL schema
Our starting point is that GraphQL's mandatory schema definition already contains all the structure needed for automatic mock generation. If you have a schema, you can get started immediately; if you don’t have an up-to-date schema, you can easily AI-generate one using the WireMock MCP server.
Here's how to create a GraphQL mock in WireMock:
- Create a new GraphQL mock API in WireMock Cloud
- Paste your GraphQL schema (or upload a schema file)
- Hit save
That's it - your mock is immediately live and serving responses.
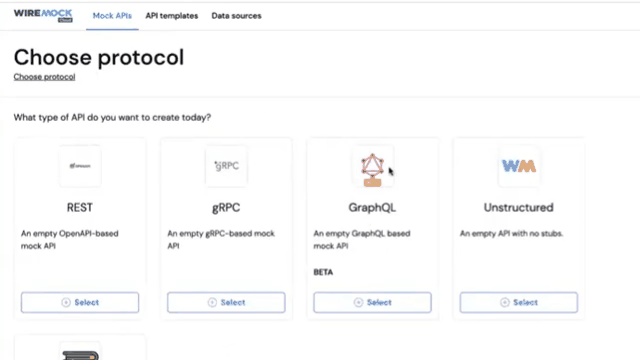
WireMock generates basic test data
WireMock analyzes your schema and generates data based on field names and GraphQL types. Fields like email get realistic email addresses, id fields receive appropriate identifiers, dates follow proper formatting, etc.
Returning to our travel app example: if you needed to display room images on a booking page but the service isn't ready, you would use the schema for the proposed room image service, create a GraphQL mock in WireMock, and simply Point your frontend to the mock URL
The frontend team can continue development with realistic data while the backend catches up. The mock generates image URLs that match the schema's pattern, letting developers build components without delays.
Refining your mock and adding advanced capabilities
Want to use something more realistic than our auto-generated dummy data? You can simply run a query against it, find the query in WireMock's request log, then convert it to a stub. You are then able to edit the stub to add data or response formats. From there, you can add more sophisticated behaviors when needed - such as using response templating to make mock data react to query variables, or adding failure states to allow for more comprehensive testing.
Update schemas locally with the WireMock CLI
Updating your mock according to schema updates is zero-touch and fully local using the WireMock's CLI. In your command line, run the following command:
wiremock push graphql <mock API ID> --file <schema file> --watch
When you push new edits to the schema via your CLI, these will automatically be reflected in WireMock Cloud - keeping your mock in sync with the latest schema without manual uploads, and enabling real-time collaboration on API design.
Learn more about GraphQL mocking in WireMock Cloud
- Watch the recent WireMock Academy video for a quick walkthrough, or the more detailed WireMock Live! session (both on Youtube)
- Read the WireMock GraphQL documentation
- Try it yourself with a free-forever WireMock Cloud account
/